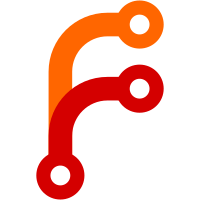
The multiplayer state object and dialogs hold a (modified) game list model, but it isn't updated when the actual game list changes. This updates the multiplayer dialogs with the new game list when it got repopulated.
74 lines
1.8 KiB
C++
74 lines
1.8 KiB
C++
// Copyright 2017 Citra Emulator Project
|
|
// Licensed under GPLv2 or any later version
|
|
// Refer to the license.txt file included.
|
|
|
|
#pragma once
|
|
|
|
#include <memory>
|
|
#include <QDialog>
|
|
#include <QSortFilterProxyModel>
|
|
#include <QStandardItemModel>
|
|
#include <QVariant>
|
|
#include "citra_qt/multiplayer/chat_room.h"
|
|
#include "citra_qt/multiplayer/validation.h"
|
|
#include "network/network.h"
|
|
|
|
namespace Ui {
|
|
class HostRoom;
|
|
}
|
|
|
|
namespace Core {
|
|
class AnnounceMultiplayerSession;
|
|
}
|
|
|
|
class ConnectionError;
|
|
class ComboBoxProxyModel;
|
|
|
|
class ChatMessage;
|
|
|
|
namespace Network::VerifyUser {
|
|
class Backend;
|
|
};
|
|
|
|
class HostRoomWindow : public QDialog {
|
|
Q_OBJECT
|
|
|
|
public:
|
|
explicit HostRoomWindow(QWidget* parent, QStandardItemModel* list,
|
|
std::shared_ptr<Core::AnnounceMultiplayerSession> session);
|
|
~HostRoomWindow();
|
|
|
|
/**
|
|
* Updates the dialog with a new game list model.
|
|
* This model should be the original model of the game list.
|
|
*/
|
|
void UpdateGameList(QStandardItemModel* list);
|
|
void RetranslateUi();
|
|
|
|
private:
|
|
void Host();
|
|
std::unique_ptr<Network::VerifyUser::Backend> CreateVerifyBackend(bool use_validation) const;
|
|
|
|
std::weak_ptr<Core::AnnounceMultiplayerSession> announce_multiplayer_session;
|
|
QStandardItemModel* game_list;
|
|
ComboBoxProxyModel* proxy;
|
|
std::unique_ptr<Ui::HostRoom> ui;
|
|
Validation validation;
|
|
};
|
|
|
|
/**
|
|
* Proxy Model for the game list combo box so we can reuse the game list model while still
|
|
* displaying the fields slightly differently
|
|
*/
|
|
class ComboBoxProxyModel : public QSortFilterProxyModel {
|
|
Q_OBJECT
|
|
|
|
public:
|
|
int columnCount(const QModelIndex& idx) const override {
|
|
return 1;
|
|
}
|
|
|
|
QVariant data(const QModelIndex& idx, int role) const override;
|
|
|
|
bool lessThan(const QModelIndex& left, const QModelIndex& right) const override;
|
|
};
|