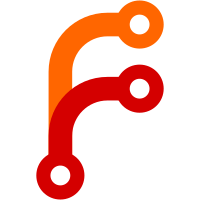
Enable cast warnings in gcc and clang and always treat warnings as errors. GetWordCount now returns std::size_t for simplicity and the word count is asserted and casted in WordCount (now called CalculateTotalWords. Silence warnings.
40 lines
866 B
C++
40 lines
866 B
C++
/* This file is part of the sirit project.
|
|
* Copyright (c) 2019 sirit
|
|
* This software may be used and distributed according to the terms of the
|
|
* 3-Clause BSD License
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <cstddef>
|
|
|
|
#include "stream.h"
|
|
|
|
namespace Sirit {
|
|
|
|
enum class OperandType { Invalid, Op, Number, String };
|
|
|
|
class Operand {
|
|
public:
|
|
explicit Operand(OperandType operand_type);
|
|
virtual ~Operand();
|
|
|
|
virtual void Fetch(Stream& stream) const = 0;
|
|
|
|
virtual std::size_t GetWordCount() const noexcept = 0;
|
|
|
|
virtual bool operator==(const Operand& other) const noexcept = 0;
|
|
|
|
bool operator!=(const Operand& other) const noexcept {
|
|
return !operator==(other);
|
|
}
|
|
|
|
bool EqualType(const Operand& other) const noexcept {
|
|
return operand_type == other.operand_type;
|
|
}
|
|
|
|
private:
|
|
OperandType operand_type;
|
|
};
|
|
|
|
} // namespace Sirit
|